In the ever-evolving landscape of web development, the quest for real-time communication has led us down many paths, but few as exciting as the WebSocket protocol, especially when combined with the power of Node.js. Imagine a world where data flows freely and instantly between the server and client, like whispers in the wind. This isn't a fantasy—it's what WebSockets in Node.js offer. Let's embark on a journey to set up a WebSocket server in Node.js, ensuring your applications speak in real-time, loud and clear.
What is Node.js and its Role in WebSocket Servers?
WebSocket Server
A WebSocket server facilitates real-time, bi-directional communication between clients and servers over a single, long-lived connection. It's like having a continuous open line on a walkie-talkie, as opposed to the traditional request-response model of HTTP. This persistent connection allows for instant data exchange, paving the way for dynamic and interactive web applications.
Node.js WebSocket Library
Node.js, with its non-blocking I/O model, is perfectly suited for handling the WebSocket's need for persistent, open connections. The ws library in Node.js is a popular choice for setting up WebSocket servers, offering a simple and efficient API to work with WebSockets. This library abstracts the complexities of the WebSocket protocol, making it accessible to developers who dream of adding real-time features to their applications.
Key Takeaway: The ws library in Node.js is your gateway to implementing efficient, real-time communication in your web applications.
How to Create and Configure a WebSocket Server in Node.js?
Setting Up WebSocket Connection
Setting up a WebSocket connection in Node.js begins with installing the ws library using npm, Node.js's package manager. Once installed, you can create a WebSocket server that listens for connections on a specified port. This setup is the first step in establishing a real-time communication channel between your server and clients.
Implementing WebSocket Server in Node.js
Implementing a WebSocket server involves initializing the WebSocket.Server object from the ws library and listening for events such as connection, message, and close. When a client connects, the server can send and receive messages through this open socket, enabling real-time data exchange. This process is akin to establishing a direct line of communication, where information flows seamlessly and instantaneously.
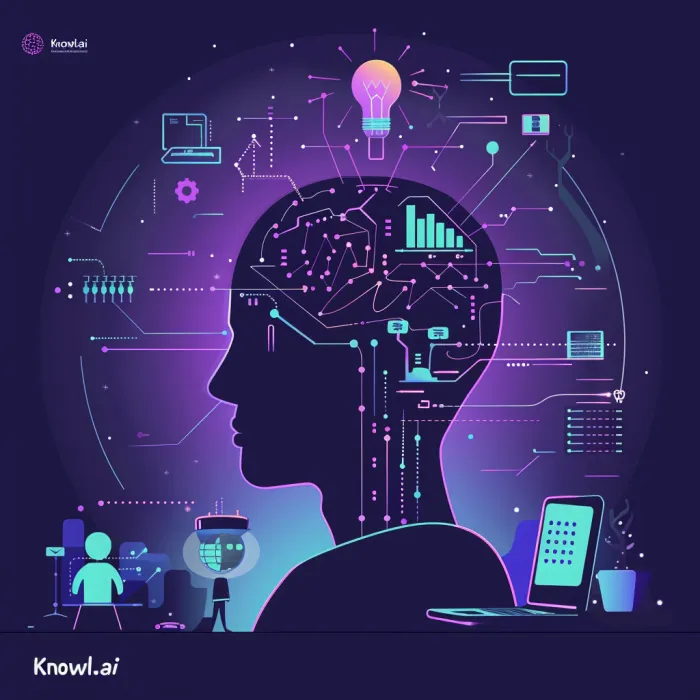
WebSocket Protocol in Node.js
The WebSocket protocol in Node.js, facilitated by the ws library, supports full-duplex communication over a single connection. This means data can be sent and received simultaneously, unlike the sequential nature of HTTP. Understanding and leveraging the WebSocket protocol allows developers to design more interactive and responsive web applications.
Key Takeaway: Mastering the WebSocket protocol in Node.js opens up a world of possibilities for creating engaging, real-time web experiences.
What are the Key Features and Functions of a WebSocket Server in Node.js?
Real-Time Communication
The hallmark of a WebSocket server in Node.js is its ability to facilitate real-time communication. This feature is crucial for applications requiring instant updates, such as chat applications, live sports updates, or real-time analytics dashboards. Real-time communication transforms user experiences, making applications feel more dynamic and alive.
Node.js WebSocket API
The Node.js WebSocket API, provided by the ws library, offers a rich set of features for managing connections, sending and receiving messages, and handling different types of data. It provides developers with the tools needed to build sophisticated real-time applications, all while maintaining a straightforward and developer-friendly interface.
WebSocket Server vs. Traditional APIs
Unlike traditional APIs that follow a request-response model, a WebSocket server maintains a persistent, open connection, allowing for instant data exchange. This fundamental difference enables applications to push updates to clients in real-time, without the need for polling or long-polling techniques, leading to more efficient network utilization and a better user experience.
Key Takeaway: Embracing the WebSocket server in Node.js can significantly enhance your application's interactivity and responsiveness, setting it apart from those using traditional APIs.
Fun Fact
Did you know that the WebSocket protocol was standardized by the IETF as RFC 6455 in 2011? It's a relatively young technology that's already making a big impact!
How to Establish Client-Server Communication with WebSocket in Node.js
Diving into the world of real-time web applications, the WebSocket protocol stands out as a superhero, enabling lightning-fast communication between a client and a server. It's like having a direct phone line that's always open, ready to transmit messages at the speed of thought. Let's embark on a journey through the realms of Node.js to set up this powerful communication channel, ensuring your applications are as lively and interactive as a bustling city square.
WebSocket Connection Establishment
Setting up a WebSocket connection in Node.js is like opening a magical portal where data flows freely and instantaneously. Using libraries such as ws or socket.io, you can create a WebSocket server that listens for connections on a specific port. When a client attempts to connect using the WebSocket protocol, the server performs a special handshake, establishing a persistent, full-duplex communication channel.
Key Takeaway: Establishing a WebSocket connection is the first step to enabling real-time communication, acting as the foundation for a responsive web application.
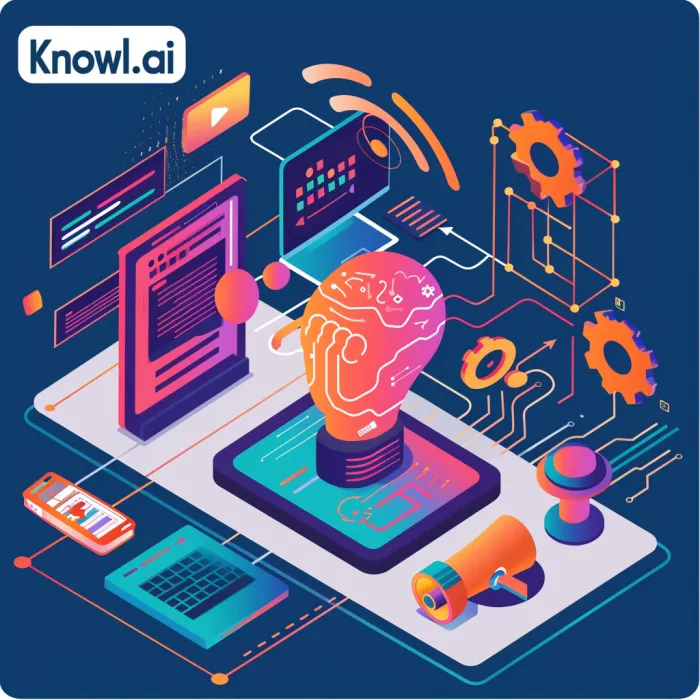
Handling Messages Between Client and Server
Once the mystical bridge between the client and server is established, the magic of real-time messaging comes to life. The server can listen for messages from the client and respond accordingly, allowing for a dynamic exchange of information. Whether it's sending updates, notifications, or live data, handling messages effectively is crucial. It's like a conversation where both parties can speak and listen simultaneously, ensuring that information is always up-to-date.
Key Takeaway: Effective message handling is vital for maintaining an engaging and interactive dialogue between the client and server.
Implementing Bidirectional Communication
The true power of WebSockets lies in their ability to support bidirectional communication, where both the client and server can initiate messages. This opens up endless possibilities for interactive applications, from live chats and gaming to collaborative editing tools. Implementing this requires careful management of the connection and ensuring that both ends can send and receive messages seamlessly.
Key Takeaway: Bidirectional communication transforms the user experience, making applications feel more alive and connected.
What Are Best Practices for Scaling and Optimizing WebSocket Servers in Node.js?
Scaling WebSockets in Node.js
As your application grows, so does the need to scale your WebSocket server to accommodate more connections. This might involve using load balancers, clustering, or even leveraging cloud services designed for real-time applications. The goal is to ensure that every user gets a smooth and responsive experience, no matter how crowded your digital city square becomes.
Key Takeaway: Scaling your WebSocket server ensures that your application can handle growth without compromising on performance.
Benchmarking WebSocket Performance in Node.js
To keep your WebSocket server running like a well-oiled machine, it's crucial to benchmark its performance. This involves testing how well your server handles various loads, identifying bottlenecks, and making necessary optimizations. Tools like WebSocket-Node or Thor can simulate thousands of connections, giving you a clear picture of your server's capabilities and limitations.
Key Takeaway: Regular benchmarking helps you stay ahead of performance issues, ensuring your WebSocket server can handle the demands of real-time communication.
Optimizing WebSocket Server in Production Environment
When moving your WebSocket server to a production environment, optimization is key. This could mean compressing data to reduce latency, implementing security measures like TLS/SSL, or optimizing your Node.js code for better performance. Every millisecond counts in real-time communication, and small optimizations can make a big difference in user experience.
Key Takeaway: Optimizing your WebSocket server for production ensures secure, efficient, and lightning-fast communication between clients and servers.
FAQ
Q: Can WebSockets replace traditional HTTP requests?
A: While WebSockets provide an efficient channel for real-time communication, they complement rather than replace traditional HTTP requests. Each has its use case, with WebSockets being the go-to for interactive, live features.
Q: Can I use WebSocket with front-end frameworks like React or Angular?
A: Absolutely! WebSockets work independently of the front-end framework, so you can easily integrate real-time functionality into your React, Angular, or Vue applications.
About Knowl.io
Introducing Knowl.io, the revolutionary AI-driven platform designed to transform how API documentation is created and maintained. Say goodbye to the painstaking process of manually updating specifications with each code change—Knowl.io does the heavy lifting for you. With seamless integration into your development workflow, Knowl.io ensures your APIdocumentation is perpetually accurate, reflecting the latest updates in your codebase without the need for manual annotations or explanations.
At the heart of Knowl.io is cutting-edge AI technology that meticulously identifies endpoints, parameters, and behaviors, crafting detailed and up-to-date API documentation with comprehensive explanations. Trust Knowl.io to elevate your documentation process, making it more efficient and reliable than ever. Ensure your developers and stakeholders always have access to the most current and coherent API documentation with Knowl.io, where innovation meets simplicity.