What are API Error Codes and How to Interpret Them?
API error codes are critical for diagnosing issues in API requests and responses, guiding developers towards effective troubleshooting.
Definition of API Error Codes
API error codes, often seen as part of the response code in an API request, are numerical or textual identifiers that specify the type of error encountered by the server while processing the API call. These codes are standardized across the web through the HTTP specification, allowing developers to quickly understand whether an error is due to client-side issues, server-side problems, or something else. Understanding these error codes is crucial for debugging and ensuring smooth communication between the client application and the server.
Importance of Understanding HTTP Status Codes
HTTP status codes are a subset of response codes specifically designed for HTTP requests. They play a vital role in API interaction, as they provide immediate feedback about the success or failure of an HTTP request. These codes are divided into categories: 1xx for informational responses, 2xx for successful responses, 3xx for redirection messages, 4xx for client errors, and 5xx for server errors. A solid grasp of HTTP status codes is essential for developers to quickly diagnose issues and understand the server's response to an API request.
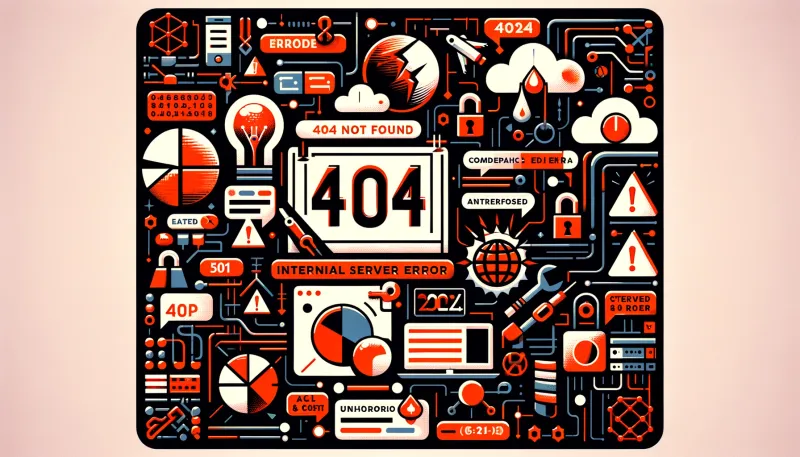
Common API Error Code Examples
- 400 Bad Request : This client error status code indicates that the server cannot process the request due to client-side issues, such as malformed request syntax or invalid request message framing.
- 401 Unauthorized : This response code means the request has not been applied because it lacks valid authentication credentials for the target resource.
- 403 Forbidden : Unlike 401, this error code signifies that the server understands the request but refuses to authorize it.
- 404 Not Found : This indicates that the server can't find the requested resource. In the context of an API, it can mean that the endpoint is incorrect or the resource does not exist.
- 500 Internal Server Error : A generic error message, given when an unexpected condition was encountered and no more specific message is suitable.
- 503 Service Unavailable : This server error response code indicates that the server is not ready to handle the request, often due to being overloaded or down for maintenance.
Each error code, whether it's a client error or a server error, provides valuable information. For instance, a 4xx error suggests that the issue lies with the request sent by the client—perhaps the API request was formed incorrectly, or the request lacked necessary information. On the other hand, a 5xx error indicates problems on the server's side, suggesting that the server failed to fulfill an apparently valid request.
Understanding these codes and the information in the response body can significantly aid in debugging API issues. It allows developers to pinpoint whether errors arise from the client's HTTP request, issues with the API's handling of the request, or problems on the server. By carefully examining the error code, the error message, and the context of the API call, developers can take specific steps to resolve the issue, whether it involves correcting the HTTP method used, adjusting the request parameters, or handling unexpected server behaviors.
How to Handle Server-side Errors in APIs?
Handling server-side errors effectively is crucial for maintaining the reliability and usability of REST APIs.
Identifying Server Errors
Server errors in REST APIs are typically indicated by 5xx status codes in the HTTP response. These codes indicate that the API server encountered an unexpected condition that prevented it from fulfilling the request. A common example is the "500 Internal Server Error," which is a generic response indicating that the server encountered an unexpected situation without a more specific message. Identifying these errors involves monitoring the response status codes and messages given in the response to understand the nature of the error.
Best Practices for Handling 5xx Status Codes
When a REST API returns a 5xx status code, it indicates that the API server has encountered an error. Best practices for handling such errors include implementing robust error logging on the server. This allows API providers to diagnose and address the underlying issue. Additionally, API endpoints should be designed to return more specific error messages with an appropriate HTTP response status code, helping the client understand the nature of the error. For example, a "503 Service Unavailable" status code should be used when the server is temporarily overloaded or under maintenance.
Dealing with Internal Configuration Errors
Internal configuration errors can lead to invalid responses from an API. Handling these errors requires thorough testing and validation of the API's configuration and environment. API providers should ensure that all components of the API, including databases and external services, are correctly configured and operational. In the event of an error, the response must include a relevant HTTP status code and, if possible, an error message that helps the client diagnose the issue. For instance, a "504 Gateway Timeout" response indicates that the API server did not receive a timely response from an upstream server.
Server-side errors are an inevitable part of working with REST APIs, but their impact can be minimized through careful planning, monitoring, and response strategies. By adhering to best practices such as detailed error logging, returning specific HTTP response status codes, and providing clear error messages, API providers can significantly improve the troubleshooting process for developers. Additionally, ensuring that the API's internal configuration is correct and that the API key and other parameters are valid can prevent many common errors. Handling server-side errors effectively not only improves the reliability of the API but also enhances the overall developer experience by making it easier to diagnose and resolve issues.
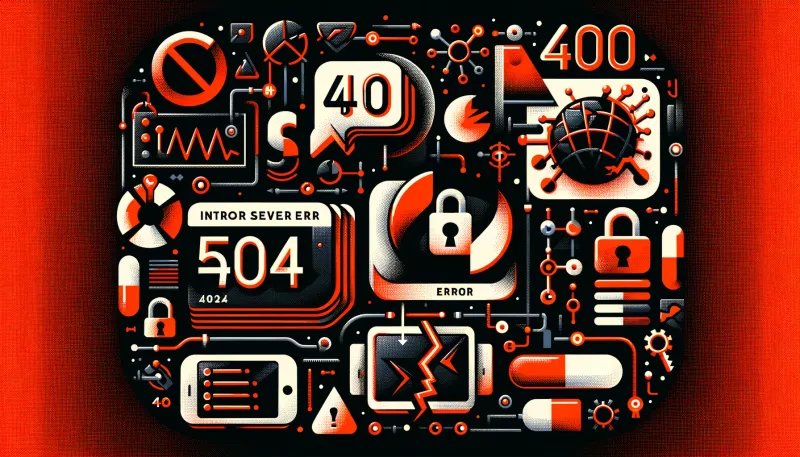
Best Practices for Managing Client-side Errors
Effectively managing client-side errors is crucial for ensuring a smooth interaction between the client and the API, enhancing user experience and debugging processes.
Understanding 4xx Status Codes
4xx status codes are specifically designed to indicate errors that stem from the client side. These responses suggest that the request contains bad syntax or cannot be fulfilled. For example, a "404 Not Found" error occurs when the requested resource could not be found on the server, and a "401 Unauthorized" response indicates that the request has not been applied because it lacks valid authentication credentials for the target resource. Understanding these codes is essential for diagnosing issues related to API requests.
Tips for Handling Client Errors in API Responses
When dealing with client errors, it's important to first check the API documentation to understand what each status code means and the expected entity of the response. For instance, a "401 Unauthorized" response may require checking if the API key is correctly included in the request header. Additionally, handling redirects properly—status codes 303 and 307 indicate that the client should make a new request to the location specified in the response's location field—is crucial for managing API calls efficiently. Implementing proper error handling in the client application can help manage these errors gracefully, improving the user experience.
Managing Invalid Responses from API Calls
Invalid responses from API calls often result from issues like internal configuration errors or incorrect request parameters. When an unexpected status code is received in response to a request, the client should have a strategy in place for handling such scenarios. This might include logging the error details, alerting the user to the issue, and providing suggestions for corrective actions. For example, a "500 Internal Server Error" indicates a problem on the server side, but a "400 Bad Request" might suggest that the client needs to modify the request before trying again. Properly managing these responses ensures that the application can handle errors robustly, maintaining functionality even when issues arise.
Client-side errors, indicated by 4xx status codes, play a critical role in the communication between a client and an API. By understanding what each status code means and implementing best practices for handling these errors, developers can create more resilient and user-friendly applications. Checking the API documentation, interpreting the response correctly, and managing invalid responses effectively are key strategies in this process. Whether it's a common "404 Not Found" error or a "401 Unauthorized" response, a well-prepared approach to handling these errors can significantly improve the overall quality of the API interaction.
Optimizing API Responses and Error Messages
Optimizing API responses and error messages is crucial for enhancing the developer experience and facilitating easier troubleshooting.
Improving Error Message Clarity
Clear error messages are essential for effective API use. When an invalid API call is made, the response should include an indication of what went wrong and, if possible, how to fix it. For example, if a response must include a `WWW-Authenticate` header due to a `401 Unauthorized` status, it should clearly state what type of authentication is required. Similarly, a `404 Not Found` status code should be accompanied by a message specifying whether the resource doesn't exist or if the endpoint is incorrect. Improving the clarity of these messages helps developers quickly understand and rectify issues.
Enhancing Response Status Communication
Standardized status codes play a significant role in API communication. Each status code allows the client to understand the general outcome of their request at a glance. For instance, a `204 No Content` response must not include a body, signaling to the client that the response has been successful but there's no content to return. On the other hand, a `307 Temporary Redirect` status code is received when the requested resource has been temporarily moved to a different URI, indicated in the response's `Location` header. Using these standardized status codes consistently helps ensure that API responses are understood universally.
Implementing Best Practices for API Error Code Handling
Best practices for handling API error codes involve a combination of clear communication and appropriate use of standardized status codes. For errors within the `5xx group of status codes`, indicating server-side issues, the response should not only return the error code but also a status message that gives insight into the error. This helps in distinguishing whether the error was caused by a server overload, a malfunction, or an unexpected condition. For client-side status error codes, it's important to guide the client on what went wrong and how to proceed. For example, if a client receives a timely response but got an invalid response due to a bad request, the error message should detail the part of the request that was invalid.
Optimizing API responses and error messages by improving error message clarity, enhancing response status communication, and implementing best practices for error code handling can significantly improve the developer experience. Clear, informative responses enable developers to quickly diagnose and fix issues, reducing frustration and increasing productivity. Whether dealing with a `404 Not Found` error, a `401 Unauthorized` response, or a `500 Internal Server Error`, providing detailed, actionable feedback is key. By ensuring that each response element avoids ambiguity and offers guidance, API providers can foster a more efficient and positive use of their APIs.
Preventative Measures and Best Practices
Implementing preventative measures and best practices in API development can significantly reduce errors and enhance security.
Security Measures in API Error Code Handling
When handling API error codes, especially those related to security such as the `401 Unauthorized` and `403 Forbidden` errors, it's crucial to provide enough information to be helpful but not so much that it exposes the system to potential security risks. For instance, a `401 error` should clearly indicate that authentication is required without revealing which part of the request was invalid, whether it was the username, password, or API key. Similarly, for a `403 error`, the response should inform the client that the request was valid but the server is refusing action. These responses should be accompanied by a generic status message that does not disclose details about the server or the underlying architecture.
Validating API Requests to Avoid Errors
Validating API requests is a critical step in preventing errors. This involves checking that the input from the client meets the expected format, type, and size before processing. Validation can help avoid a range of issues, from `4xx error` codes, which indicate client-side problems, to `5xx status codes`, which signal server-side errors. For example, thorough validation can prevent a `429 Too Many Requests` response by ensuring that each client adheres to rate limits set by the API. By validating requests, APIs can ensure that only valid and authorized requests are processed, reducing the likelihood of errors and improving the overall security posture.
Utilizing API Key Authentication for Error Prevention
API key authentication is a simple yet effective way to control access and prevent errors associated with unauthorized use of the API. By requiring an API key with each request, servers can quickly authenticate clients before processing their requests. This method helps in avoiding unauthorized access, which could lead to `401 Unauthorized` errors, and ensures that clients are correctly identified and have the permissions needed to use the API. Furthermore, API key authentication can be used to monitor and control how the API is used, preventing abuse that could result in a `429 Too Many Requests` response or even trigger a `5xx error` due to overload.
Conclusion
Preventative measures and best practices in API development and error handling are essential for maintaining a secure, efficient, and user-friendly API. By implementing security measures that balance informativeness with caution, validating API requests to ensure they meet predefined criteria, and utilizing API key authentication to control access, developers can mitigate many common issues that lead to error responses. These strategies not only improve the security and reliability of the API but also enhance the experience for end-users by providing clearer, more actionable error messages and reducing the likelihood of encountering errors in the first place.
Knowl.io
Introducing Knowl.io, the revolutionary AI-driven platform designed to transform how API documentation is created and maintained. Say goodbye to the painstaking process of manually updating specifications with each code change—Knowl.io does the heavy lifting for you. With seamless integration into your development workflow, Knowl.io ensures your API documentation is perpetually accurate, reflecting the latest updates in your codebase without the need for manual annotations or explanations.
At the heart of Knowl.io is cutting-edge AI technology that meticulously identifies endpoints, parameters, and behaviors, crafting detailed and up-to-date API documentation with comprehensive explanations. Trust Knowl.io to elevate your documentation process, making it more efficient and reliable than ever. Ensure your developers and stakeholders always have access to the most current and coherent API documentation with Knowl.io, where innovation meets simplicity.